You can easily validate a phone number in Vue.js without using any external library. Below I’ve written a code which you may follow to learn how to do it without using any NPM package:
Here’s my App.vue (single component) which does the validation:
<template>
<div id="app">
<label for="phoneNumber"> Enter phone number </label>
<input
name="phoneNumber"
:class="{ valid: isValidPhoneNumber, invalid: !isValidPhoneNumber }"
v-model="phoneNumber"
type="text"
@keyup="validatePhoneNumber"
/>
<div class="invalid-warning" v-if="!isValidPhoneNumber">
Invalid phone number!
</div>
<div class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
phoneNumber: "",
isValidPhoneNumber: true,
};
},
methods: {
validatePhoneNumber() {
const validationRegex = /^\d{10}$/;
if (this.phoneNumber.match(validationRegex)) {
this.isValidPhoneNumber = true;
} else {
this.isValidPhoneNumber = false;
}
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
padding: 24px;
}
.np-credits {
font-size: 12px;
margin-top: 12px;
color: #4b4b4b;
}
label {
font-size: 20px;
display: block;
margin: 10px auto;
font-weight: 600;
}
input {
display: block;
outline: none;
border: 2px solid #eee;
font-size: 20px;
padding: 10px;
background: #eee;
border-radius: 6px;
}
.invalid {
border: 2px solid red;
}
.valid {
border: 2px solid green;
}
.invalid-warning {
margin: 10px auto;
color: red;
}
</style>
Code language: HTML, XML (xml)
In the above code, I’m using two reactive states:
- phoneNumber: To v-model with the user input number
- isValidPhoneNumber: To specify whether or not the entered number is valid
Whenever the user presses a key, the validatePhoneNumber method will be fired. This method will check whether the entered phone number matches the validationRegex format or not.
You can choose and create whatever regex you want to validate the phone number with.
This method will set isValidPhoneNumber to true if user enters a valid number. Otherwise the method will set its value to false.
Finally, we’ve displayed the “Invalid phone number!” message if isValidPhoneNumber value is false.
Here are two views for two states of the validation:
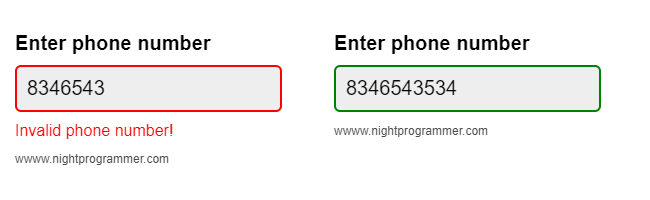