In the previous article, we learned how we can render a polygon using the Data Layer in Google Maps JavaScript API v3 in Vue.js.
In this example, we’ll have a look at how we can toggle the visibility of the polygon with the click of a button to hide and show it.
Here’s a constants.js file to contain the coordinates of the polygon:
export const polygonCoords = [
{ lat: 28.4173106, lng: 77.0406078 },
{ lat: 28.4014569, lng: 77.0450281 },
{ lat: 28.406553, lng: 77.0587181 },
{ lat: 28.4208963, lng: 77.0517658 },
{ lat: 28.4173106, lng: 77.0406078 }
];
Code language: JavaScript (javascript)
Here’s the code we need to implement the toggle visibility functionality:
<template>
<div>
<div class="header-margins">
<div style="margin-top: 20px">
<button @click="togglePolygonVisibility">
{{ isPolygonVisible ? "Hide" : "Show" }} polygon
</button>
</div>
</div>
<div id="data-layers-map" class="map-margins"></div>
</div>
</template>
<script>
import loadGoogleMapsApi from "load-google-maps-api";
import { gMapsApiKey, polygonCoords } from "./../constants";
export default {
name: "ToggleDataLayerPolygonVisibility",
data() {
return {
map: null,
isPolygonVisible: true,
};
},
created() {
this.polygonCoords = polygonCoords;
},
mounted() {
loadGoogleMapsApi({
key: gMapsApiKey,
libraries: ["drawing", "geometry"],
}).then(async () => {
const mapZoom = 14;
const { google } = window;
const mapOptions = {
zoom: mapZoom,
mapTypeId: google.maps.MapTypeId.HYBRID,
center: new google.maps.LatLng({ lat: 23, lng: 57 }),
mapTypeControl: true,
streetViewControl: false,
mapTypeControlOptions: {
position: google.maps.ControlPosition.BOTTOM_LEFT,
},
};
this.map = new google.maps.Map(
document.getElementById("data-layers-map"),
mapOptions
);
const coordinates = this.polygonCoords;
const tempBounds = new google.maps.LatLngBounds();
for (let j = 0; j < coordinates.length; j++) {
const x = {
lat: coordinates[j].lat,
lng: coordinates[j].lng,
};
const BoundLatLng = new google.maps.LatLng({
lat: parseFloat(x.lat),
lng: parseFloat(x.lng),
});
tempBounds.extend(BoundLatLng);
}
const centroid = tempBounds.getCenter();
this.map.data.add({
geometry: new google.maps.Data.Polygon([coordinates]),
});
this.map.setCenter(centroid);
});
},
methods: {
togglePolygonVisibility() {
this.isPolygonVisible = !this.isPolygonVisible;
this.map.data.setStyle({
visible: this.isPolygonVisible,
});
},
},
};
</script>
<style scoped>
.header-margins {
margin-left: 40px;
margin-top: 20px;
}
.map-margins {
height: 400px;
width: 600px;
margin: 10px 40px;
}
button {
background-color: hsla(160, 100%, 37%, 1);
color: #fff;
margin-right: 4px;
}
</style>
Code language: HTML, XML (xml)
In the code above, we’ve created a method through lines 75-80. We’re using a reactive state named isPolygonVisible. We’re using this state to update the visibility of the polygon. data.setStyle({visible: Boolean}) is the method we’re using to toggle the visibility.
Here’s what the above code would look like in action:
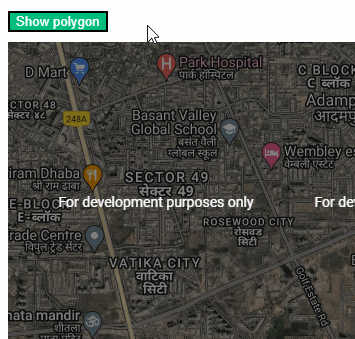
You can find a working version of the above code from my repos here: