Vue.js comes with the v-for directive to render any sort of list with ease. Earlier, I’ve also written an article on how you can define your own custom directives in Vue.js. Here in this article we’ll see how you can render a list of records, passed as a prop using the createElement and render() function. Here’s the code for main.js file:
import Vue from "vue";
import App from "./App.vue";
Vue.component("colors", {
props: ["colorsList"],
render(createElement) {
if (this.colorsList.length) {
return createElement(
"ul",
this.colorsList.map((c) => {
return createElement("li", c);
})
);
} else {
return createElement("p", "Empty list!");
}
}
});
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App)
}).$mount("#app");
Code language: JavaScript (javascript)
As you can see in the above code, I’ve created a component named colors. I’ve then passed in a prop named colorsList. Then, with the callback function createElement inside the render function, I’ve created an element ul. This element will have the list of li elements with the value of c. Here, c denotes each of the item of the colorsList prop.
You can then use colors component in any component and pass in the values for the prop colorsList. Here’s my App.vue file which does the same thing:
<template>
<div id="app">
<h3>Colors list</h3>
<colors :colorsList="myColors" />
<small>wwww.nightprogrammer.com</small>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
myColors: ["Red", "Blue", "Green", "Yellow"],
};
},
};
</script>
<style>
body {
font-family: Arial, Helvetica, sans-serif;
}
</style>
Code language: HTML, XML (xml)
The output of the above code would look like:
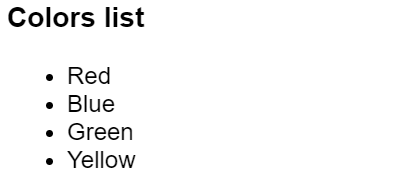