Using conditional statements and rendering something to the DOM with Vue.js is pretty easy. If you were to do the same thing using vanilla JavaScript, that would be a whole different story. Vue.js can check for a truthy-statement during compilation and render it to the DOM. What’s more interesting is the fact that, if the statement becomes false because of a user action or an external API-request, it can update the DOM instantly. Without having to do anything at all.
Let’s try to understand this with few examples. Simply go to jsfiddle.com and write the following code:
In the HTML window:
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<div id="app">
<p>
My name is Gautam.
</p>
<p>
My name is Tommy.
</p>
</div>
Code language: HTML, XML (xml)
In the above code, first we are importing the Vue.js library in our application and then using an HTML id called “app” to bind it with the Vue.js instance.
Now write the following code in the JavaScript window:
new Vue({
el: ‘#app’,
data: {
}
});
Code language: CSS (css)
In this code, you’re only creating the Vue instance without actually passing any data to the Vue.js template. Note that there’s a blank object called “data” defined inside the Vue.js instance. This is where you keep the current template data, unless you’re getting the data from Vuex (Vue.js store) or a global state, of course.
After writing this code, hit the Run button. If everything goes well, you should see the following in the output window:
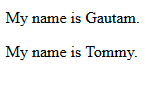
That’s right. Nothing fancy. In most of the applications, however, you do not show everything to the user all the time. For example, you may want to show some error message only when a certain condition fails. Say, for instance, a user is trying to sign-up on your application using a username which is already being used by some other user. It’s pretty easy to handle such situations in Vue.js with the help of conditional-statements. In our case, let’s start with the v-if condition.
How to use the v-if condition?
Modify the JavaScript code in JSFiddle as follows:
new Vue({
el: ‘#app’,
data: {
showGautam: true,
showTommy: false
}
});
Code language: CSS (css)
You’ll notice that I’ve added two variables called showGautam and showTommy, with default values of true and false assigned respectively. These are the values that Vue.js compiler checks during rendering the DOM. It then decides which block of elements to keep in the HTML, and which are to be avoided. To do that, modify the two paragraph elements in the HTML window as follows:
<p v-if=”showGautam”>
My name is Gautam.
</p>
<p v-if=”showTommy”>
My name is Tommy.
</p>
Code language: HTML, XML (xml)
In the above code, I’ve added two v-if elements, called v-if=”showGautam” and v-if=”showTommy”. There’s something interesting about these conditions.
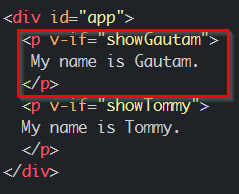
Whatever the v-if conditions are wrapped up with, they work directly on the whole element. You’ll understand that better with the next example. But right now, it’s sufficient to understand that whatever is returned by the right side evaluation of the v-if statement is taken into consideration.
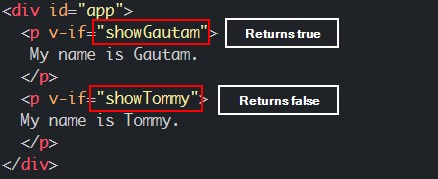
Here, in the right side of the evaluation, we have a variable called showGautam. It is simply a variable declared in the data object, with a default value of true. That means, true is being returned to the v-if statement in the first case. Therefore, the whole HTML p element is rendered to the browser window, with the inner text that says “My name is Gautam”.
In the second p element however, the showTommy variable returns a false to the v-if statement. Therefore, the whole element is destroyed during the compile time only. And therefore “My name is Tommy” is not shown. It is important to understand that the whole element is removed from the HTML structure, and not just hidden! If you right click on your browser window and try to see the source code, you’ll see something like this:
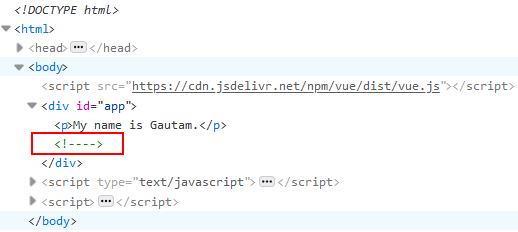
See, there’s only one <p> element present. But, you’ll notice there’s also an empty comment <!—->. This is Vue.js telling you something. It’s saying that there was supposed to be an element here, but it was destroyed because some condition failed to meet. And that condition was returning of true. This leads to some interesting questions, like:
Can Vue.js only have true and false for v-if conditions?
Well, yes and no. The final result is always a boolean, either true or false. However, if you talk about the types of data that can be passed to the variables in the data objects, there’s more. If the modify the JavaScript code as follows, it’ll still work the same:
new Vue({
el: ‘#app’,
data: {
showGautam: 1,
showTommy: 0
}
});
Code language: CSS (css)
I’ve replaced true with 1 and false with 0. If you run the code now, you’ll still get the same result. Does that mean true can be replaced with 1 and false can be replaced with 0?
In the first paragraph of the article, I said Vue.js checks for truthy-statements. It doesn’t have to be true only. It must, however, evaluate to true in the end. Any number, positive or negative. Fractional, or whole. Except for 0, or 0 followed by a decimal and then more zeroes, it’ll always return true. For example, 1, 1.234, 3.14, -10, -22.7, 0.0001 will all return true. While, 0 or 0.0 or 0.00 will return false. undefined and null will also return false.
It doesn’t just end here, you can even use strings to check for truthy conditions. For example, the following code will also show “My name is Gautam” and remove “My name is Tommy”:
new Vue({
el: ‘#app’,
data: {
showGautam: “Yes, show his name”,
showTommy: “”
}
});
Code language: CSS (css)
This is simply because, showGautam is now a non-emtpy variable. It contains a string of characters. Therefore, it evaluates to true. However, showTommy contains an empty string, therefore it has no value, and evaluates to false.
How to use v-if condition with && and || operators?
Let’s dig a bit deeper to see what else v-if can do for us. v-if can actually compare for more than just one condition. It can combine multiple conditions together. Let’s try to understand that from the following example. Modify the JS code as follows:
new Vue({
el: ‘#app’,
data: {
showGautam: true,
showTommy: true,
showTommyReally: false
}
});
Code language: CSS (css)
In the code above, you’ve added one more variable named showTommyReally and assigned it to false. Now, modify the HTML as follows:
<p v-if="showGautam">
My name is Gautam.
</p>
<p v-if="showTommy && showTommyReally">
My name is Tommy.
</p>
Code language: HTML, XML (xml)
Now run the code, and you show still just see the first paragraph. But why did the v-if condition in the second paragraph failed?
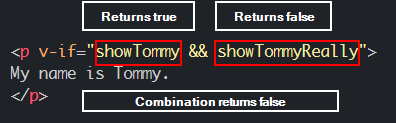
This is because we’re using the “AND operator” (&&) in the condition check. It means, both the conditions declared before and after the AND operator must return true. If any one, or both fail to return true, the entire statement fails and returns false to the v-if. Now, let’s change the value of showTomReally to true and see what happens. Modify the code as follows:
data: {
showGautam: true,
showTommy: true,
showTommyReally: true
}
Code language: CSS (css)
And run the code, you’ll see this on the screen:
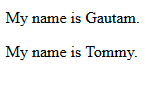
Similarly, you can also use the OR operator (||). In case of OR operator, if any of the variables declared before and after the OR operator returns true, then true is returned to the whole v-if statement. To check this, again modify the value of showTommyReally back to false. And modify the HTML as follows:
<p v-if="showGautam">
My name is Gautam.
</p>
<p v-if="showTommy || showTommyReally">
My name is Tommy.
</p>
Code language: HTML, XML (xml)
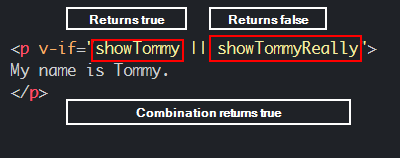
In the above code, there’s an OR operator (||). The whole combination returns true because one of the variables returned true (showTommy, in this case). Therefore, the whole v-if statement takes in true and displays the element.
Okay, enough talking about the v-if condition. Just like any programming language, there’s an else statement as well. Vue.js calls it the v-else statement. There are times when you want to show the user one of the two options. That’s when v-else comes in handy. Let’s have a look.
How to use the v-else condition?
Let’s try to understand it with an example. Suppose you have a website with some Signing up functionality. But, you only want people above 18 to sign up. In that case, if someone below the age of 18 tries to sign up, you want to show some warning message. Go to jsfiddle.com and paste the following code inside the JavaScript window:
new Vue({
el: ‘#app’,
data: {
userAge: 21
}
});
Code language: CSS (css)
You’ve declared a variable named userAge with a default value of 21.
And then, paste the following code in the HTML window:
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<div id="app">
<p v-if="userAge >= 18">
You can sign up!
</p>
<p v-else>
Sorry you can’t sign up!
</p>
</div>
Code language: HTML, XML (xml)
Here, in the first p element, the user will see “You can sign up!” if only the userAge has a value greater than or equal to 18. Otherwise the second p element “Sorry you can’t sign up” would be shown, because it’s wrapped with the v-else statement. Now go ahead and run the code by clicking on Run and you should see the following output:
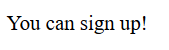
You are seeing that message because the user is 21, hence above 18. Now change the value of 21 to something smaller than 18, like 14. Run the code again and you should now see “Sorry you can’t sign up!”. It’s that easy. One thing worth noting down here is that when there is a v-if statement with a v-else statement and nothing else, one of the two will surely work. Both of them won’t work together at any case.
How to use the v-else-if condition?
Suppose the user is above 16 year old. In that case, you want to show the user that he can sign up soon. Let’s modify the HTML a little more, and make the following changes:
<div id="app">
<p v-if="userAge >= 18">
You can sign up!
</p>
<p v-else-if="userAge >= 16">
You will be able to sign up soon!
</p>
<p v-else>
Sorry you can’t sign up!
</p>
</div>
Code language: HTML, XML (xml)
In the code above, I’ve added an intermediate condition, called v-else-if. This condition will evaluate to true if the right side of the condition returns true. In the JavaScript code, change the user’s age from 21 to 16 and run the code. Now you should see “You will be able to sign up soon!”. This is because the first v-if condition failed (userAge is not greater than or equal to 18). Then Vue.js checked the second condition. Here it found that the userAge is indeed greater than or equal to 16. So it displays the message written inside the p element. Once Vue.js finds any of the condition true, it doesn’t check anymore. Therefore, the v-else statement theoretically not only returns false, but is not checked at all.
How to hide an element from the DOM without actually detaching it using v-show?
So far, we’ve learned that if a condition fails, the whole element is removed from the DOM and is replaced by an empty comment (<!—->). However, there might be times you wouldn’t want to remove an element altogether from the DOM and only hide it temporarily. In that case, instead of using v-if, you can just use v-show. v-if and v-show work almost the same way, that is, it evaluates the truthiness of the right side of the expression. With a difference being that, v-show hides the element from the DOM, but stays in the DOM. It uses the CSS property (display:none) to hide the element. There’s one downside of using v-show over v-if, though. That is, you can’t use v-else or v-else-if with v-show.
How to display multiple elements conditionally?
Up until now, we’ve been only showing single elements. But how would you group certain number of elements? Say, for instance, you want to show a heading and a paragraph if a certain condition is matched. And you’d show an error if it is not matched. So would it work if you combine them in a div? Let’s check that out.
Modify the HTML code as follows:
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<div id="app">
<div v-if="userAge >= 18">
<h1>You can sign up!</h1>
<p>We’d love to have you onboard!</p>
</div>
<p v-else-if="userAge >= 16">
You will be able to sign up soon!
</p>
<p v-else>
Sorry you can’t sign up!
</p>
</div>
Code language: HTML, XML (xml)
In the above code, we’re grouping the h1 element and the first p element using a div, and wrapping it with v-if. Also, change the JavaScript code as follows:
new Vue({
el: ‘#app’,
data: {
userAge: 20
}
});
Code language: CSS (css)
As per the code goes, the userAge is now 20, therefore, the first v-if condition stands true. You’ll see the following output:
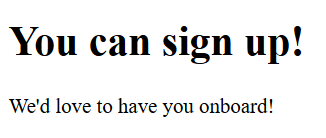
So, it is possible to group elements using <div> tags. However, I’d recommend you to avoid using it. Instead, in Vue.js, you can use an alternative called the <template> tag. This tag doesn’t bring you the CSS properties that a <div> element does. So, the modified code should look like:
<div id="app">
<template v-if="userAge >= 18">
<h1>You can sign up!</h1>
<p>We’d love to have you onboard!</p>
</template>
<p v-else-if="userAge >= 16">
You will be able to sign up soon!
</p>
<p v-else>
Sorry you can’t sign up!
</p>
</div>
Code language: HTML, XML (xml)