In this tutorial, we’ll have a look at how we can change URL / src of an image by clicking on button in Vue.js. Here’s the code we’d need to implement the functionality:
<template>
<div>
<h3>Vue 3 change image URL with button click</h3>
<button @click="showPreviousImage">Previous</button>
<button @click="showNextImage">Next</button>
<div class="np-ht">
<img :src="imageUrl" />
</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
imageId: 1,
};
},
computed: {
imageUrl() {
return `https://picsum.photos/id/${this.imageId}/500/400`;
},
},
methods: {
showPreviousImage() {
if (this.imageId > 1) this.imageId--;
},
showNextImage() {
this.imageId++;
},
},
};
</script>
Code language: HTML, XML (xml)
In the above code, we’ve conditionally bind a src tag of the img element with the reactive state imageUrl on line 7. We’ve initiated its default value to 1, on line 17. Then we’ve placed two buttons on lines 4 and 5 and bound them with the functions showPreviousImage and showNextImage respectively.
We have a computed property named imageUrl dependent on reactive state imageId on line 21. It means that whenever the value of imageId changes, the computed property also gets updated. And whenever we update this property, the img element re-renders itself, thus showing the next image.
Here’s what the above code would look like in action:
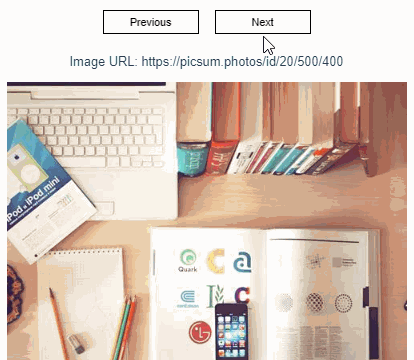
You can find a working version of the above example from my repo links below: