In this tutorial, we’ll have a look at how we can shake the input field box when a user types something invalid.
First, we create a class that has an animation of shaking the input field with the help of CSS animation keyframes.
We then add this class to the invalid field for a few seconds when we want to shake the invalid field.
Here’s a code example to demonstrate the same:
<script setup>
import { ref } from 'vue';
const isInputInvalid = ref(false);
const inputPasswordValue = ref('');
const checkInputValidity = () => {
isInputInvalid.value = inputPasswordValue.value.length < 8
if(isInputInvalid.value) {
shakeInputField()
} else {
document.querySelector('.input-password').classList.remove('invalid-password')
alert('Success!')
}
}
const shakeInputField = () => {
document.querySelector('.input-password').classList.add('invalid-password')
setTimeout(() => {
document.querySelector('.input-password').classList.remove('invalid-password')
}, 1000)
}
</script>
<template>
<div>
<h2>
Enter password
</h2>
<input
type="password"
class="input-password"
v-model="inputPasswordValue" />
<p v-if="isInputInvalid">
Password should be at least 8 characters long!
</p>
<div>
<button @click="checkInputValidity">Submit</button>
</div>
</div>
</template>
<style scoped>
.input-password {
font-size: 16px;
letter-spacing: 3px;
padding: 4px 6px;
outline: none;
border: 1px solid #000;
}
.invalid-password {
border: 1px solid red;
animation-name: shake-input;
animation-iteration-count: infinite;
animation-duration: 0.2s;
}
@keyframes shake-input {
0% {
transform: translateX(-10px)
}
25% {
transform: translateX(0px)
}
50% {
transform: translateX(10px)
}
75% {
transform: translateX(0px)
}
100% {
transform: translateX(-10px)
}
}
</style>
Code language: HTML, XML (xml)
In the above code, we have a reactive state named isInputInvalid on line 4. This is to store whether or not the entered input valid is valid or not at any given moment.
Then on line 7, we have another method named checkInputValidaty(). This will check and assign the value of isInputInvalid. If it finds that the value is true, then it’ll call the shakeInputField() method on line 18.
This method will first add the ‘invalid-password’ class to the input field and then remove the same class after 1 second.
This is how the above code should look in action:
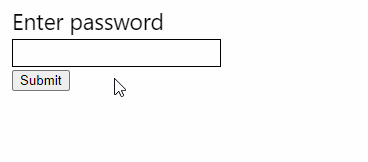
You can find a working version of the above code from my repo links below: