Many times we may need to mask the user input with hyphens, spaces, periods, etc. This is useful when we want to accept some information in a predefined way, such as credit card numbers, credit card security code, telephone numbers, etc.
It means that, when the user enters some number, it’ll be shown as 1234-5678-9012-3456, however the actual accepted value would be 1234567890123456 only.
This can be easily achieved in Vue.js using the v-mask library.
First of all, install the library with:
npm i v-mask
Now, we need to register the library in the main.js file for Vue to use it globally:
import Vue from "vue";
import App from "./App.vue";
import VueMask from "v-mask";
Vue.use(VueMask);
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App)
}).$mount("#app");
Code language: JavaScript (javascript)
Once done, we can now use it in our app:
<template>
<div id="app">
<input
type="text"
placeholder="Enter number"
v-mask="'#### - #### - #### - ####'"
v-model="userInputValue"
/>
<p>Masked input: {{ userInputValue }}</p>
<h3>Parsed value: {{ parsedInputValue }}</h3>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
userInputValue: "",
};
},
computed: {
parsedInputValue() {
let matchedInputs = this.userInputValue.match(/\d+/g);
let parsedString = "";
if (matchedInputs && matchedInputs.length) {
matchedInputs.forEach((m) => {
parsedString += m;
});
}
return parsedString;
},
},
};
</script>
<style>
input {
font-size: 18px;
padding: 4px 8px;
border: 2px solid rgb(98, 0, 255);
background: rgb(234, 221, 255);
outline: none;
}
</style>
Code language: HTML, XML (xml)
As we can see in the above code, we’ve used the v-mask directive on line 6. We then v-model the user input to the userInputValue reactive state. We’ve then shown the masked value and the parsed value of the user input on lines 9 and 10 respectively. We’ve computed the parsedInputValue by extracting all the numbers from the user input.
This is what the above code would look like in action:
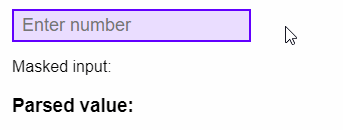
You can find a working version of the above code from my repos below: