YouTube allows iframes to be used for embedding their videos in your web page. But those iframes come with the limitations of not having direct controls / event listeners. Vue.js has multiple libraries which can tackle that issue. Here, in this article, I’ll be showing one such library. You’ll see how you can directly access the play, pause, stop, playing etc states of the video and add custom functionality to it.
First of all, you need to install these two libraries:
npm install vue-youtube-embed vue-play
- vue-youtube-embed will help you embed a YouTube video.
- vue-play will help you have explicit controls over the video.
You need to register the component in your main.js file as follows:
import Vue from "vue";
import App from "./App.vue";
import VueYouTubeEmbed from "vue-youtube-embed";
Vue.use(VueYouTubeEmbed);
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App)
}).$mount("#app");
Code language: JavaScript (javascript)
Next up, you can now embed the video like this:
<template>
<div id="app">
<div>
<div class="np-credits">www.nightprogrammer.com</div>
<section>
<youtube
:video-id="videoId"
:player-width="500"
:player-height="300"
@ready="ready"
@playing="playing"
></youtube>
</section>
<div class="np-ib np-button" @click="play">Play</div>
<div class="np-ib np-button" @click="pause">Pause</div>
<div class="np-ib np-button" @click="stop">Stop</div>
</div>
</div>
</template>
<script>
import { getIdFromURL } from "vue-youtube-embed";
let videoId = getIdFromURL("https://www.youtube.com/watch?v=24C8r8JupYY");
export default {
name: "App",
data() {
return {
videoId,
};
},
methods: {
ready(event) {
this.player = event.target;
},
playing(event) {
console.log("playing");
},
change() {
//this.videoId = "use another video id";
},
stop() {
this.player.stopVideo();
},
pause() {
this.player.pauseVideo();
console.log("paused");
},
play() {
this.player.playVideo();
console.log("paused");
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
margin: 15px 10px;
}
.np-ib {
display: inline-block;
}
.np-button {
margin-top: 10px;
background: #0051ff;
color: #ffffff;
width: 80px;
margin-right: 10px;
text-align: center;
padding: 4px 8px;
border-radius: 4px;
cursor: pointer;
transition: all 0.3s;
}
.np-button:hover {
background: #4e86ff;
transition: all 0.3s;
}
.np-credits {
font-size: 12px;
padding-bottom: 14px;
}
</style>
Code language: HTML, XML (xml)
You need to import getIdFromUrl from vue-youtube-embed. This method will help you get the videoId as I did on line 23. You can also define multiple event triggers inside methods as I’ve done:
this.player.playVideo(): To play the video. You can also define some custom functionality here.
this.player.pauseVideo(): To pause the currently playing video
this.player.stopVideo(): To stop the video from playing
playing(): You can trigger it to add some functionality during the video playback every time
You can of course, bind these methods to any HTML elements you want. I’ve bind them with custom buttons on line 14, 15, and 16.
Here’s a preview of the above code render:
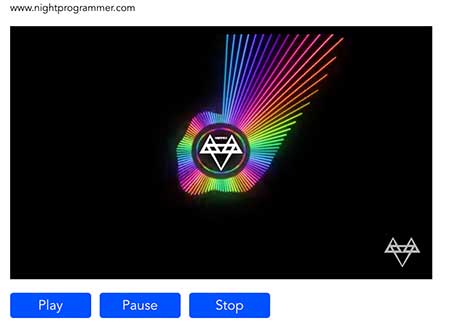
Here you can get the working version of the demo: