Using v-if and v-show directives is probably the most popular way to hide or show a component. These directives in Vue actually gains more popularity as it is missing in React. While you can of course toggle display between components or some tab content using v-if or v-show, Vue.js actually provides in-built and better ways to do it properly. You can do so by using the v-bind:is directive.
I’m going to show how that works by creating three tab contents named about, profile and photos. I’ll name these components about-tab, profile-tab and photos-tab respectively. Here’s my main.js file doing the same:
import Vue from "vue";
import App from "./App.vue";
Vue.config.productionTip = false;
Vue.component("about-tab", {
template: "<div>About me</div>"
});
Vue.component("profile-tab", {
template: "<div>My profile</div>"
});
Vue.component("photos-tab", {
template: "<div>My photos</div>"
});
new Vue({
render: (h) => h(App)
}).$mount("#app");
Code language: JavaScript (javascript)
And here’s my App.vue file using these components on demand:
<template>
<div id="app">
<button @click="selectedTab = 'About'">About</button>
<button @click="selectedTab = 'Profile'">Profile</button>
<button @click="selectedTab = 'Photos'">Photos</button>
<div class="tab-content">
<component v-bind:is="selectedTabComponent"></component>
</div>
<div class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
selectedTab: "about",
};
},
computed: {
selectedTabComponent() {
return `${this.selectedTab.toLowerCase()}-tab`;
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
padding: 24px;
}
button {
background-color: #004cbe;
color: #ffffff;
border: 0px;
border-radius: 6px;
padding: 6px 20px;
margin-right: 1em;
cursor: pointer;
transition: all 0.3s;
}
button:hover {
background-color: #2f79e7;
transition: all 0.3s;
}
.tab-content {
margin-top: 20px;
background: #eee;
padding: 12px;
border-radius: 4px;
}
.np-credits {
font-size: 12px;
margin-top: 12px;
color: #4b4b4b;
}
</style>
Code language: HTML, XML (xml)
As you can see in the above file, I’ve placed three buttons, each of which would update the selectedTab property. The selectedTabComponent computed property would then attach a -tab to the component name. So, for ‘About’, it’d get changed to ‘about-tab’. The component then get’s activated using the v-bind:is=”about-tab” as shown on line number 8. The component on line 8 is used to dynamically show a component. Just as we did right now.
The above code when compiled would produce the following output:
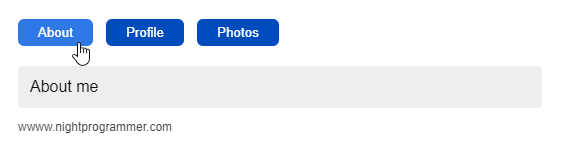
You can find a working demo of the above code from my repo links below: