Sometimes doing a simple task (like changing a text to uppercase or lowercase) can turn tedious and take away a large amount of time. Thanks to the watch property of Vue.js, making changes to a state has become so easy. With the watch, it’s possible to modify the existing value of a state upon its own modification. It’s also important to be careful while using the watch, any wrong implementation could easily lead to an infinite rendering loop.
Now, let’s have a quick look at how to auto-convert input text upon its change. Here’s the template and script code for the functionality:
<template>
<div id="app">
<div style="height: 20px">
<small v-show="originalUserInputValueObservable">
www.nightprvogrammer.com
</small>
</div>
<div class="np-input-text--value_holder">
<input
class="np-input-text--value"
type="text"
v-model="originalUserInputValueObservable"
/>
</div>
<div
class="np-input-text--preview np-input-text--preview_changed"
v-if="userInputValueUppercase"
>
Uppercased: <strong>{{ userInputValueUppercase }}</strong>
</div>
<div
class="np-input-text--preview np-input-text--preview_changed"
v-if="userInputValueLowercase"
>
Lowercased: <strong>{{ userInputValueLowercase }}</strong>
</div>
<div
class="np-input-text--preview np-input-text--preview_changed"
v-if="originalUserInputValueObservable"
>
Original: <strong>{{ originalUserInputValueObservable }}</strong>
</div>
<div
v-if="!originalUserInputValueObservable"
class="np-input-text--preview np-input-text--preview_initial"
>
{{ intialPlaceHolderText }}
</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
originalUserInputValueObservable: "",
userInputValueUppercase: "",
userInputValueLowercase: "",
intialPlaceHolderText: "Start typing to see conversions ...",
};
},
watch: {
originalUserInputValueObservable() {
this.userInputValueUppercase = this.originalUserInputValueObservable.toUpperCase();
this.userInputValueLowercase = this.originalUserInputValueObservable.toLowerCase();
},
},
beforeDestroy() {
this.resetInputValueStates();
},
beforeCreate() {
this.resetInputValueStates();
},
methods: {
resetInputValuesInitialStates() {
this.originalUserInputValueObservable = "";
this.userInputValueUppercase = "";
this.userInputValueLowercase = "";
},
},
};
</script>
Code language: HTML, XML (xml)
As you can see, I’m using local state originalUserInputValueObservable as a watch property. Whenever a user types something, the watcher will look for a change in its value. If it detects a change in value, I’ve updated the states containing uppercase and lowercase conversions. These two states are userInputValueUppercase and userInputValueLowercase. I’ve rendered these changed values on line 19, 25 and 31.
This is how the view should look like:
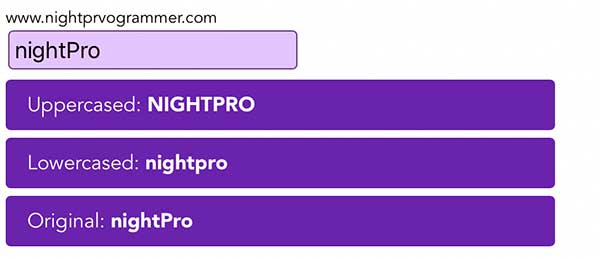
I’ve also added some CSS for styling:
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
.np-input-text--value_holder {
margin-bottom: 6px;
}
.np-input-text--value {
padding: 4px;
font-size: 18px;
background: rgb(228, 196, 255);
border: 1px solid rgb(107, 66, 128);
border-radius: 4px;
color: rgb(0, 0, 0);
outline: none;
}
.np-input-text--preview {
color: #fff;
border-radius: 4px;
margin-top: 4px;
margin-bottom: 6px;
padding: 8px 16px;
max-width: 400px;
}
.np-input-text--preview_changed {
border: 1px solid rgb(106, 35, 172);
background: rgb(106, 35, 172);
word-break: break-all;
}
.np-input-text--preview_initial {
border: 1px solid rgb(172, 35, 99);
background: rgb(172, 35, 99);
}
</style>
Code language: HTML, XML (xml)
You can find a working copy of the above code from my repos below: