Vue.js provides multiple ways to filter some data. Some of these ways are through common mixins, component based methods, some helper functions, etc. But if you need to filter some data, or chain response of one filter on another there’s more. You can easily do that with the pipe filters in Vue templates. For instance, you can first find out the length of a string for a given string. And then tell whether the length is even or odd based on the result of the previous filter. Here’s an example to demonstrate the idea. Here’s the App.vue file:
// App.vue
<template>
<div id="app">
<h2>Enter some string:</h2>
<div>
<input type="text" v-model="inputString" />
</div>
<div>
<h4>String length: {{ inputString | getStringLength }}</h4>
<h4>
Even string length:
{{ inputString | getStringLength | isStringLengthEven }}
</h4>
</div>
<div class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
inputString: "",
};
},
filters: {
isStringLengthEven(stringLength) {
return stringLength % 2 === 0;
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
padding: 24px;
}
.np-credits {
font-size: 12px;
}
</style>
Code language: HTML, XML (xml)
In the above code, isStringLengthEven is a local filter which simply tells whether a passed number is a even or odd number. This filter is bound to the scope of the component App.vue.
And here’s main.js file:
// main.js
import Vue from "vue";
import App from "./App.vue";
Vue.config.productionTip = false;
Vue.filter("getStringLength", function (str) {
if (!str) return 0;
return str.length;
});
new Vue({
render: (h) => h(App)
}).$mount("#app");
Code language: JavaScript (javascript)
In the above code, getStringLength is a global filter which can be used anywhere directly in the Vue templates of any component.
The result of the above code would look like:
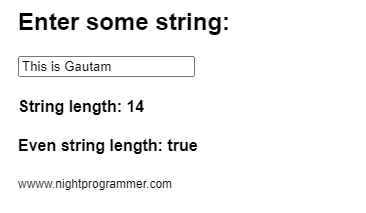
You can find a working demo of the above code from my repos here: