In this article, we will learn how to use the Vue 3 Composition API to get the day of the week from a date object.
Code example
<script setup>
import { ref, onMounted } from 'vue'
const day = ref('')
onMounted(() => {
day.value = getDayOfWeek()
})
const getDayOfWeek = () => {
const weekDays = [
'Sunday',
'Monday',
'Tuesday',
'Wednesday',
'Thursday',
'Friday',
'Saturday',
]
return weekDays[new Date().getDay()]
}
</script>
<template>
<div>
<div id="np-container">
<p>Today is {{ day }}</p>
</div>
</div>
</template>
<style scoped>
#np-container {
padding: 10px;
}
</style>
Code language: HTML, XML (xml)
Explanation
First, we need to import the ref state handler and the onMounted hook.
import { ref, onMounted } from 'vue'
Code language: JavaScript (javascript)
Next, we will create a day variable as a ref and initialize its value to an empty string (by default).
const day = ref('')
Code language: JavaScript (javascript)
Then, we will use the onMounted hook to call a function getDayOfWeek that will set the value of the day variable to the current day of the week.
onMounted(() => {
day.value = getDayOfWeek()
})
Code language: JavaScript (javascript)
The getDayOfWeek function is defined as follows:
const getDayOfWeek = () => {
const weekDays = [
'Sunday',
'Monday',
'Tuesday',
'Wednesday',
'Thursday',
'Friday',
'Saturday',
]
return weekDays[new Date().getDay()]
}
Code language: JavaScript (javascript)
The function creates an array of weekdays and returns the current day of the week by using the getDay method on a new Date object and accessing the corresponding element in the weekDays array.
In the template, we will use the day variable to display the current day of the week:
<template>
<div>
<div id="np-container">
<p>Today is {{ day }}</p>
</div>
</div>
</template>
Code language: HTML, XML (xml)
Finally, we can add some styling to the container element using scoped CSS:
<style scoped>
#np-container {
padding: 10px;
}
</style>
Code language: HTML, XML (xml)
If everything works well, you should be able to see the day of the week as shown below:
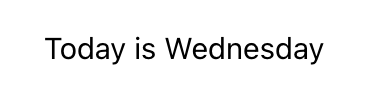