In the previous post, we saw how we can extract all the numbers from a string in JavaScript. In this post, we’ll have a look at how we can extract all the numbers from a user input value and use it as needed.
<template>
<div id="app">
<input
type="text"
placeholder="Type something..."
v-model="userInputValue"
/>
<p>User input: {{ userInputValue }}</p>
<h3>Parsed value: {{ parsedInputValue }}</h3>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
userInputValue: "",
};
},
computed: {
parsedInputValue() {
let str = this.userInputValue;
let matches = str.match(/\d+/g);
let matchedString = "";
if (matches && matches.length) {
matches.forEach((m) => {
matchedString += m;
});
}
return matchedString;
},
},
};
</script>
Code language: HTML, XML (xml)
In the above code, we’ve created a reactive state named userInputValue. We’ve v-model this state with the user input. That is the value of this state changes as the user types.
We’ve also created a computed property named parsedInputValue. This property depends on the value of userInputValue and returns the extracted number from the input. You can read the previous article to know in detail how the method works.
This is how the above code would look like in execution:
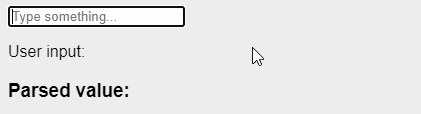
You can find a working version of the above code from my repose below: