You can easily create a drag-and-drop list where you can drag one item and drop it in a different position in within the list. The fastest and easiest way to achieve the functionality is by using the vuedraggable library.
First you need to install the npm package by running:
npm install vuedraggable -s
Once the package is installed, you can now use it anywhere in your application.
I’ve created a list of data which I’m going to use as an example. I’ve kept the array list in a file called items.js:
const items = [
{
id: 1,
name: "Gautam"
},
{
id: 2,
name: "Night"
},
{
id: 3,
name: "Joe"
},
{
id: 4,
name: "Chandler"
},
{
id: 5,
name: "Ross"
},
{
id: 6,
name: "Rachael"
},
{
id: 7,
name: "Monica"
}
];
export default items;
Code language: JavaScript (javascript)
And here’s my App.vue file demonstrating the example:
<template>
<div id="app">
<h2>Draggable list</h2>
<draggable group="words" :list="itemsList" @change="showDragged">
<div
class="np-item np-item-unselectable"
v-for="item in itemsList"
:key="item.id"
>
{{ item.name }}
</div>
</draggable>
<div class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
import draggable from "vuedraggable";
import items from "./items";
export default {
name: "App",
components: {
draggable,
},
data() {
return {
itemsList: items,
};
},
methods: {
showDragged(e) {
console.log(`Moved element ${e.moved.element.name}`);
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
padding: 24px;
}
.np-credits {
font-size: 12px;
margin-top: 12px;
color: #4b4b4b;
}
.np-item {
padding: 4px;
border: 1px solid #eee;
border-radius: 4px;
background: #eee;
cursor: pointer;
margin: 6px 0;
}
.np-item:active {
background: rgb(168, 168, 168);
}
.np-item-unselectable {
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
</style>
Code language: HTML, XML (xml)
In the code above, I’ve imported the list of data I’ve previously mentioned on line 19. I’ve then copied the data to the itemsList reactive property on line 28. I’ve used the vuedraggble component on line 4 and passed in the itemsList to the items prop. The list items are now draggable. Whenever you drag an item, an event is passed and you can catch that using the @change event listener. I’ve bind a local method named showDragged to this @change event listener.
I’ve console logged the moved element name. Alternatively, you can access the entire moved item and use it if required. The moved item would also keep reference of the oldIndex (where the item is moved from) and newIndex (where the item is moved to). The moved object would look something like this:
{
element: {
id: 1
name: "Gautam"
}
oldIndex: 0
newIndex: 1
}
Code language: CSS (css)
Here’s how the above code would look like in action:
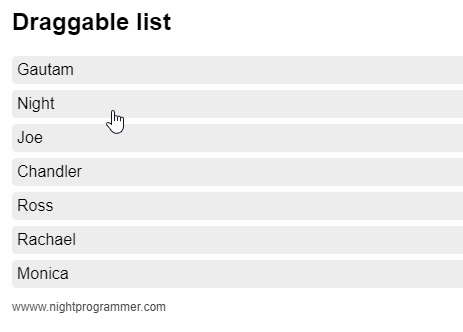