Caching can greatly help with your application performance. As far as template data, you can easily cache the rendered content using v-once if it doesn’t need to be changed or updated. Here’s an example on how you can cache some content:
<template>
<div id="app">
<div v-once>Hello there!</div>
<p v-once>
Please click the Increase button to increase counter value and Decrease
button to decrease counter value.
</p>
<div>
<h3>Counter: {{ counter }}</h3>
<button @click="increaseCounter">Increase</button>
<button @click="decreaseCounter">Decrease</button>
</div>
<div v-once class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
counter: 0,
};
},
methods: {
increaseCounter() {
this.counter++;
},
decreaseCounter() {
this.counter--;
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
padding: 24px;
}
p {
max-width: 400px;
}
button {
background-color: #004cbe;
color: #ffffff;
border: 0px;
border-radius: 6px;
padding: 6px 20px;
margin-right: 1em;
cursor: pointer;
transition: all 0.3s;
}
button:hover {
background-color: #2f79e7;
transition: all 0.3s;
}
.np-credits {
font-size: 12px;
margin-top: 12px;
color: #4b4b4b;
}
</style>
Code language: HTML, XML (xml)
In the above code, I’ve used v-once on line 3, 4 and 13. It means that, these parts of the code won’t get updated or re-rendered even if the component is re-rendered. Notice that I’ve placed two buttons named Increase and Decrease. These two buttons would essentially increase or decrease the counter values when clicked. I’ve then shown the exact value of the counter on line 9. Now, if the place a v-once on the h2 element, you’d no more see the updated values even if you click the Increase or Decrease buttons.
<h3 v-once>Counter: {{ counter }}</h3>
Code language: HTML, XML (xml)
So, when using the v-once directive, you need to be extra careful and only use it on static content. Also, if you’re using in a project of involving multiple developers, make sure everyone knows how it works.
Here’s an output of the above mentioned code:
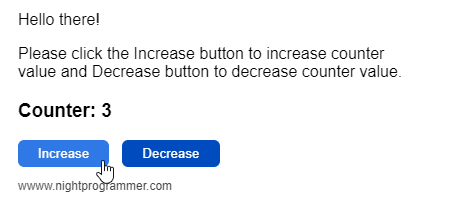
If you have any doubts or suggestions, let me know in the comments below. You can find a working copy of the above code from my repo links below: