Slots are a great way to pass some fixed data from the parent to the child component. We can then arrange the position and appearance of the data wherever and however we want.
Here we demonstrate the idea with a parent component passing in some title and paragraph data to a child component as slots.
Using slots in templates
<script setup>
import ChildComponent from './ChildComponent.vue';
</script>
<template>
<div>
<ChildComponent>
<template #title>
Some title passed via slot
</template>
<template #paragraph>
Some paragraph passed via slot
</template>
</ChildComponent>
</div>
</template>
Code language: HTML, XML (xml)
In the code above, we’ve created two named slots named title and paragraph. We’ve then passed in some textual data to those slots. Here’s the ChildComponent which receives the slot data:
<template>
<div>
<h1>Child component</h1>
<h2 class="c-title">
<slot name="title"/>
</h2>
<p class="c-paragraph">
<slot name="paragraph" />
</p>
</div>
</template>
<style scoped>
.c-title {
color:red
}
.c-paragraph {
color: blue
}
</style>
Code language: HTML, XML (xml)
The output of the above code would look like this:
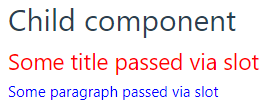
Using and accessing slots programmatically in scripts
In the Options API, we could access slots using the this.$slots.<slotname>() syntax. However in the Composition API, we can access slots using the useSlots composable as shown below:
<script setup>
import { useSlots } from 'vue'
const slots = useSlots()
//this.$slots.title()
console.log(slots.title())
</script>
Code language: HTML, XML (xml)
If you have any doubts or suggestions, let me know in the comments below!