Svelte allows us to explicitly show an element if the array to be looped is found empty. It’s a feature that’s exclusively available to Svelte among other SPA frameworks.
Let’s have a look at an example for a better understanding. In this example, we have a reactive list (initially empty) with name displayedUsers.
We have another state containing a list of users by the name users.
We use a local function toggleListData to reset or refill the list data in displayedUsers from users state.
//CardComponent.svelte
<script>
export let name
export let email
export let index
</script>
<div>
<div class="crd">
<div class="crd-header">
{index + 1}. {name}
</div>
<div class="crd-content">
{email}
</div>
</div>
</div>
Code language: HTML, XML (xml)
//App.svelte
<script>
import CardComponent from './CardComponent.svelte'
let users = [
{
id: 1,
name: 'Gautam',
email: 'gautam@example.com',
},
{
id: 2,
name: 'Sam',
email: 'sam@example.com',
},
{
id: 3,
name: 'Tim',
email: 'tim@example.com',
},
]
let displayedUsers = []
const toggleListData = () => {
displayedUsers = displayedUsers.length === 0 ? users : []
}
</script>
<div>
<button on:click="{toggleListData}">
{displayedUsers.length ? 'Clear data' : 'Generate data'}
</button>
{#each displayedUsers as user, index}
<CardComponent name={user.name} email={user.email} {index} (user.id) />
{:else}
<p>No users found!</p>
{/each}
</div>
Code language: HTML, XML (xml)
You’d notice that in the above file, we have an :else block on line 37. Although this block is directly inside the :each loop, it’ll check whether the list is empty or not. Whenever dislayedUsers array is set to empty, the element inside the else block gets displayed. Therefore, if we clear the displayedUsers array, we see a message ‘No users found!’.
Here’s what the above code would look like:
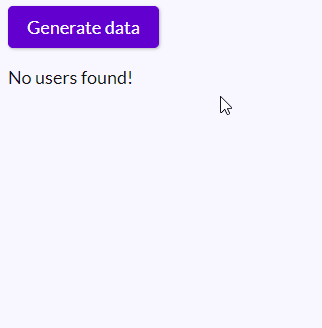