In the previous example, we learned how we can do two-way data binding in Svelte by using the :on event listener.
However, it required us two steps to bind a reactive state to the input value. For instance, in the previous example, we had this input field:
<input type="text" value={name} on:input="{setName}"/>
Code language: HTML, XML (xml)
- value={name} would set the reactive value of name to the value of the input
- on:input=”{setName}” would set the user input value to the reactive state name
Svelte actually gives us an option to do this in a single step. We can directly bind the value of the user input to the name reactive state and also to the input field value. We can do this with the following syntax:
<input type="text" bind:value={name} />
Code language: HTML, XML (xml)
This saves us from creating a function that would save the user input value to the reactive value. However, this should be used with caution and only wherever applicable. If we want to achieve some more functionality or computation other than binding the value directly, it is not the preferred way. For complex scenarios, it is still better to create our own event listeners and bind them with the functions.
Here’s the complete snippet:
<script>
let name = '';
$: lowercasedName = name.toLowerCase()
</script>
<div>
<p>Name in lower case: <span class="lc">{lowercasedName}</span></p>
<input type="text" bind:value={name} />
</div>
<style>
.lc {
font-weight: 800;
color: blue;
}
</style>
Code language: HTML, XML (xml)
And this is what the above code would look like in action:
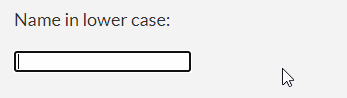