There can be situations where you want to merge the key-value pairs of two JavaScript objects into one. You can do it in multiple ways. Here I’m going to show two popular ways to do it. One, a classic approach by looping over the values of each of the keys in an Object. And another, using the spread operator given in JavaScript.
Let’s say I have two objects name keyboard and electronic. Now I want to merge their values into a single object. Please keep in mind that only the unique key: value pairs will be merged in either approach.
const keyboard = {
type: 'Electronic device',
weightInKg: 1,
}
const electronic = {
isBiodegradable: false,
isPortable: true,
}
let newElectronicKeyboard = {}
for (key in keyboard) {
newElectronicKeyboard[`${key}`] = keyboard[key]
}
for (key in electronic) {
newElectronicKeyboard[`${key}`] = electronic[key]
}
console.log(newElectronicKeyboard)
Code language: JavaScript (javascript)
You can also get the same result using the spread operator as follows:
const keyboard = {
type: 'Electronic device',
weightInKg: 1,
}
const electronic = {
isBiodegradable: false,
isPortable: true,
}
const newElectronicKeyboard = { ...keyboard, ...electronic }
console.log(newElectronicKeyboard)
Code language: JavaScript (javascript)
This is how the merged object (output) should look like:
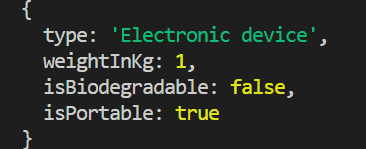
Now, let’s see what happens if any of the objects contain properties with same key names:
const keyboard = {
type: 'Electronic device',
weightInKg: 1,
}
const electronic = {
isBiodegradable: false,
weightInKg: 2,
}
const newElectronicKeyboard = { ...keyboard, ...electronic }
console.log(newElectronicKeyboard)
Code language: JavaScript (javascript)
You’ll now get the following output instead:
{ type: 'Electronic device', weightInKg: 2, isBiodegradable: false }
Code language: CSS (css)
This is because of the fact that a JavaScript object can only contain unique keys. Therefore, the key-value pair in keyboard object (weightInKg: 1) got replaced by the key-value pair in electronic object (weightInKg: 2).
If you have any doubts, sound off in the comments below!