There are multiple ways to scroll to a target element in Vue.js. Here in this tutorial, we’re going to see how we can scroll to an element having an id. We’ll assign id’s to elements dynamically, and then scroll to the required one using scrollIntoView() method. Let’s have a look at how we do that:
<script setup>
import { ref } from 'vue';
const targetId = ref('200')
const scrollToElement = () => {
const el = document.getElementById(targetId.value);
if (el) {
el.scrollIntoView();
}
}
</script>
<template>
<div>
<button @click="scrollToElement">Scroll to {{targetId}}</button>
<input type="text" v-model="targetId" />
<ul>
<li v-for="n of 500" :key="n" :id="n">
{{ n }}
</li>
</ul>
</div>
</template>
<style scoped>
ul {
padding-lefT: 0;
}
li {
background: #eee !important;
list-style-type: none;
margin-bottom: 1em;
margin-top: 1em;
padding: 4px;
}
</style>
Code language: HTML, XML (xml)
In the above code, we initiate a reactive state with the name targetId having the value ‘200’. We then dynamically create a list of items on line 20.
We also have an input field on line 18 to target an element with an id (just for testing).
On line 17, we have a button to trigger the scrollToElement method. This method will get the value of the targetId, and if any element is found with that id, it’ll scroll into that.
This is how it should look like:
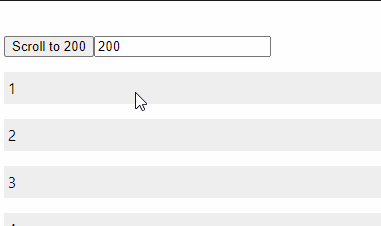
You can find a working version of the above code from my repo links below: