In the previous article, we saw how we can check if input contains any special characters in Vue.js. In this article, we’ll see how to check whether an input contains numbers.
Here’s the example code that demonstrates the same:
<template>
<div id="app">
<input
type="text"
placeholder="Type something..."
v-model="inputValue"
@keyup="inputContainsNumbers"
/>
<p>
Contains number:
<span v-if="inputContainsNumber">✅ True</span>
<span v-else>❌ False</span>
</p>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
inputValue: null,
inputContainsNumber: false,
};
},
methods: {
inputContainsNumbers() {
const numbers = /\d/;
this.inputContainsNumber = numbers.test(this.inputValue);
},
},
};
</script>
Code language: HTML, XML (xml)
In the above code, we have a method named inputContainsNumbers which checks whether or not input contains any digit. This boolean value is then set to the inputContainsNumber reactive state.
Based on the value of inputContainsNumber, we show a message in the template. We show whether it’s true or false.
Here’s what it looks like in execution:
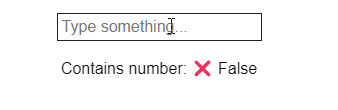
You can find a working version of the above code from repos below: