Earlier we’ve seen how we can create editable Polygons and Polygons with labels using the Google Maps JavaScript API v3. Google Maps can not only create shapes that can be used as a location parameter. But we also create polygons with data layers using the Google Maps JavaScript API. The main advantage of using the Data Layer is that we can keep some data along with Features on the map, and use them as we need. Data Layer Features are also significantly better in performance as compared to shapes in Google Maps.
Here’s an example I’ve created to show how we can create a polygon using google.maps.Data.Polygon.
Here’s the component having the Google Map mounted file:
<template>
<div>
<div id="data-layers-map" class="map-margins"></div>
</div>
</template>
<script>
import loadGoogleMapsApi from "load-google-maps-api";
import { gMapsApiKey } from "./../constants";
export default {
data() {
return {
map: null,
};
},
mounted() {
loadGoogleMapsApi({
key: gMapsApiKey,
libraries: ["drawing", "geometry"],
}).then(async () => {
const mapZoom = 14;
const { google } = window;
const mapOptions = {
zoom: mapZoom,
mapTypeId: google.maps.MapTypeId.HYBRID,
center: new google.maps.LatLng({ lat: 23, lng: 57 }),
mapTypeControl: true,
streetViewControl: false,
mapTypeControlOptions: {
position: google.maps.ControlPosition.BOTTOM_LEFT,
},
};
this.map = new google.maps.Map(
document.getElementById("data-layers-map"),
mapOptions
);
const polygonCoords = [
{ lat: 28.4173106, lng: 77.0406078 },
{ lat: 28.4014569, lng: 77.0450281 },
{ lat: 28.406553, lng: 77.0587181 },
{ lat: 28.4208963, lng: 77.0517658 },
{ lat: 28.4173106, lng: 77.0406078 },
];
const tempBounds = new google.maps.LatLngBounds();
for (let j = 0; j < polygonCoords.length; j++) {
const x = {
lat: polygonCoords[j].lat,
lng: polygonCoords[j].lng,
};
const BoundLatLng = new google.maps.LatLng({
lat: parseFloat(x.lat),
lng: parseFloat(x.lng),
});
tempBounds.extend(BoundLatLng);
}
const centroid = tempBounds.getCenter();
this.map.data.add({
geometry: new google.maps.Data.Polygon([polygonCoords]),
});
this.map.setCenter(centroid);
});
},
};
</script>
Code language: HTML, XML (xml)
As we can see, through lines 62-64, we created a polygon and added it to the data object of the map instance. We’ve created the Polygon as a geometry object.
The above code would generate the following polygon on map:
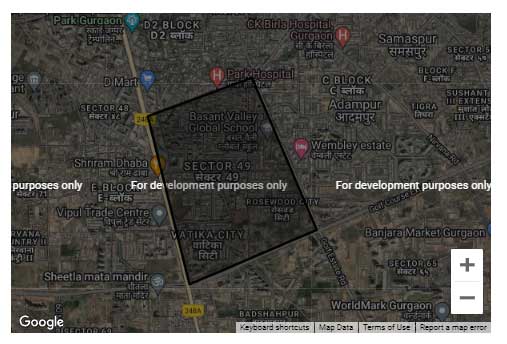
You can find a working version of the above example from my repos here: