There are plenty of ways to export data to CSV, XLS, TXT, or whichever format we want in Vue.js. One of the most significant advantages of using Vue.js is its compatibility with Vanilla JS libraries. It can perform really well with Vanilla JS libraries without any customization out of the box.
Let’s have a look at how we can export JSON data to Excel (xls) format in Vue.js.
Project setup
First, we need to install an NPM package called export-from-json. We can fire up the terminal and enter the following command:
npm install export-from-json -s
Code language: JavaScript (javascript)
After the package and its dependencies are installed, we can now use the library. Now, we’re going to create a helper method to parse the data. I’m going to call it excel-parser.js:
import exportFromJSON from "export-from-json";
export const excelParser = () => {
function exportDataFromJSON(data, newFileName, fileExportType) {
if (!data) return;
try {
const fileName = newFileName || "exported-data";
const exportType = exportFromJSON.types[fileExportType || "xls"];
exportFromJSON({ data, fileName, exportType });
} catch (e) {
throw new Error("Parsing failed!");
}
}
return {
exportDataFromJSON
};
};
Code language: JavaScript (javascript)
As we can see, we first imported the library at line 1. We’ve then created a closure named exportDataFromJSON. Of course, we can call it whatever we want.
We’ve then passed three parameters to it:
- data: The JSON data (Array) which we need to parse
- newFileName: We can pass a file name to it by generating a random file name if we want. Or we’ll just use ‘export-data’ name by default in case nothing is passed.
- fileExportType: Here we can pass the export file type (xls, csv, txt, HTML, etc).
We’ve finally called exportFromJSON at line 9 which generates the required file.
Here’s the sample-data.js file content. Of course, in a real-world scenario, we’re more likely to fetch some data from a remote server instead.
export const sampleData = [
{ id: 1, name: "Night", email: "nightprogrammer95@gmail.com" },
{ id: 2, name: "Gautam", email: "mailgautam@test.com" },
{ id: 3, name: "Alex", email: "xalex@testmail.com" },
{ id: 4, name: "Lina", email: "lina@mail.com" },
{ id: 5, name: "Peter", email: "peter.me@test.com" }
];
Code language: JavaScript (javascript)
And here we have the component (App.vue) to use the above helper:
<template>
<div id="app">
<h2>Export data to Excel example in Vue.js</h2>
<button @click="exportData">Download file</button>
<div class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
import { excelParser } from "./excel-parser";
import { sampleData } from "./sample-data";
export default {
name: "App",
data() {
return {
listData: sampleData,
};
},
methods: {
exportData() {
excelParser().exportDataFromJSON(this.listData, null, null);
},
},
};
</script>
Code language: HTML, XML (xml)
In the above file, we’ve first imported the sample data and excelParser helper we just created.
We’ve then stored the sample data in the listData state. Finally, we’ve called the exportData method (line 21) on the button click on line 4.
Demo
Here’s what it should look like:
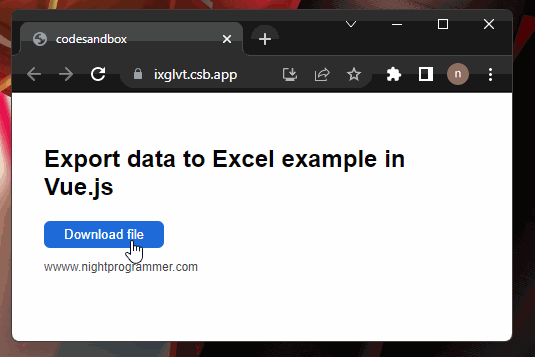
You can find a working version of the above code from my repos here: