We can observe any change in a reactive state in Vue.js using the watch property.
watch has changed a bit from Options API to Composition API.
In Options API, watch was a property which we’d use like this:
watch: {
someVariable(newValue, oldValue) {
//do stuff
}
}
Code language: JavaScript (javascript)
However, in the Composition API, watch is a method that we can use in this way:
watch(() => someVariable, (newValue, oldValue) => {
//do stuff
})
Code language: JavaScript (javascript)
Let’s take an example using the watch method. In this example, we can enter some text input, be it a name. We can then display a message depending on the length of the entered text. If the text length is less than 3, we’d show ‘Name too short!’, otherwise we’d show ‘Good name length!’
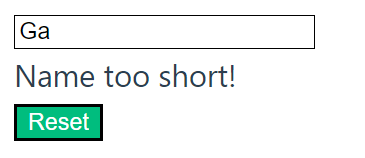
Here’s the code for the above scenario:
<template>
<div>
<input name="name" v-model="inputData.name" type="text"
placeholder="Enter name" />
<h3 v-if="inputData.nameLengthMessage">
{{inputData.nameLengthMessage}}
</h3>
<div v-if="inputData.name" class="buttons-container">
<button @click="clearName">Reset</button>
</div>
</div>
</template>
<script setup>
import { reactive, watch } from "vue";
const inputData = reactive({
name: '',
nameLengthMessage: ''
})
watch(() => inputData.name, (newName, oldName) => {
if(newName.length < 3) {
inputData.nameLengthMessage = 'Name too short!'
} else {
inputData.nameLengthMessage = 'Good name length!'
}
})
const clearName = () => (inputData.name = "");
</script>
Code language: HTML, XML (xml)
As we can see in the above code, we first import the watch method from vue at line 15. We’ve then used it on line 22. We’re watching the property inputData.name and checking for the newName value. We can get the newly entered name through the newName and the old state from oldName. In this case, we don’t really need the oldName. Now, whenever the newName length is less than 3, we update the value of inputData.nameLengthMessage with ‘Name too short!’. Otherwise, we’re updating it with ‘Good name length!’.