Composition API has several advantages over the Options API. I’ve written an article on what they are. Here in this article, I’m showing an example on how we can convert a component using Options API to Composition API.
Consider the following component taking a user input. Here we can enter a name and when we click on the Greet button, we see our name as an alert. We can reset the user input with the Reset button.
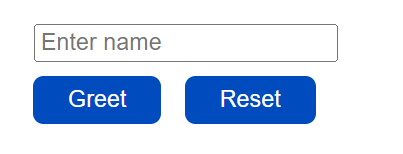
<template>
<div>
<input v-model="name" type="text" placeholder="Enter name" />
<div class="buttons-container">
<button @click="greetName">Greet</button>
<button @click="clearName">Reset</button>
</div>
</div>
</template>
Code language: HTML, XML (xml)
And here’s the script code using the Options API:
<script>
export default {
data() {
return {
name: "",
};
},
methods: {
greetName() {
alert(`Hello ${this.name}!`);
},
clearName() {
this.name = "";
},
},
};
</script>
Code language: HTML, XML (xml)
We can convert the above code into Composition API with the following steps:
- Use setup keword with script tag: script setup
- Use ref() for defining reactive properties
- Use <property-name>.value to read or write the property
The above code in Composition API would look like this:
<script setup>
import { ref } from "vue";
const name = ref("");
const greetName = () => alert(`Hello ${name.value}!`);
const clearName = () => name.value = "";
</script>
Code language: HTML, XML (xml)
As we can see, the lines of code has reduced from 17 to 7! And this is just a small component. For projects with large components, we’ll be able to see a significant decrease in codebase size.