The ability to pass a functional child component to a parent component is one of the most powerful features provided by React.js. Here in this tutorial, I’ll show you how to do that in an easy way. Here’s the App.js file of the React application:
import "./styles.css";
import ParentComponent from "./ParentComponent";
import ChildComponent from "./ChildComponent";
export default function App() {
const message = "Test message in child component";
return (
<div className="App">
<ParentComponent>
<ChildComponent message={message} />
</ParentComponent>
</div>
);
}
Code language: JavaScript (javascript)
In the above code, you’d notice that I’ve imported both ParentComponent and ChildComponent from their respective files. I’ve then passed a message to the message prop of the ChildComponent. I’ll discuss both the components one by one in a bit.
Here’s the ParentComponent.js:
import React from "react";
const ParentComponent = (props) => {
return (
<div className="parent-wrapper">
<h3>Parent header</h3>
<div>{props.children}</div>
</div>
);
};
export default ParentComponent;
Code language: JavaScript (javascript)
The ChildComponent passed through the ParentComponent is accessible inside the ParentComponent only by using the children (line 7) props. You can access whatever component you pass through the ParentComponent directly inside it and place it wherever you want to. Therefore, this ParentComponent is free to take in and use any child component.
And here’s the ChildComponent.js:
import React from "react";
const ChildComponent = (props) => {
return <div className="child-wrapper">{props.message}</div>;
};
export default ChildComponent;
Code language: JavaScript (javascript)
The above code is for the ChildComponent which I passed earlier to the ParentComponent. This component is also independent on its own. It means that you can use this component either as a child component or an individual component without passing it as a child component.
I’ve also added some CSS styles to better distinguish the components separately:
.App {
font-family: sans-serif;
text-align: center;
}
.parent-wrapper {
border: 1px solid rgb(255, 0, 0);
background-color: rgb(255, 213, 213);
margin: 10px 0px;
}
.child-wrapper {
margin: 4px;
padding: 20px;
border: 1px solid rgb(0, 0, 255);
background-color: rgb(218, 218, 255);
}
Code language: CSS (css)
If everything works well, you should see something like this on the screen:
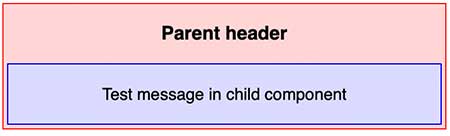