There may be occasions when you’d want user to enter a username with valid alphabets and numbers only (alphaNumeric). You might also want to show a warning if the entered characters doesn’t fall in any of the category. Here in this example, I’ll show you how to do that:
Here’s the template code I’ve written for the same:
<template>
<div id="app">
<div>Enter anything, only alphabets and numbers will be accepted:</div>
<div>
<input
class="NPInputValueField"
type="text"
name="inputValue"
v-model="inputValue"
@keydown="checkKeyDownAlphaNumeric($event)"
/>
</div>
<div v-if="inputValue" class="NPInputResponseContainer">
Validated value: <strong>{{ inputValue }}</strong>
</div>
<div v-if="ignoredValue" class="NPInputResponseContainer">
Ignored character: <strong>{{ ignoredValue }}</strong>
</div>
<div style="margin-top: 22px">
<small>www.nightprogrammer.com</small>
</div>
</div>
</template>
Code language: HTML, XML (xml)
You’d notice that I’ve called a function named checkKeyDownAlphaNumeric() on every key down from the keyboard. Then on line 14 and 17, I’ve shown the validated and ignored entered input.
Here I’ve written the logic script:
<script>
export default {
name: "App",
data() {
return {
inputValue: "",
ignoredValue: "",
};
},
methods: {
checkKeyDownAlphaNumeric(event) {
if (!/[a-zA-Z0-9\s]/.test(event.key)) {
this.ignoredValue = event.key ? event.key : "";
event.preventDefault();
}
},
},
};
</script>
Code language: HTML, XML (xml)
As you can see, I’ve tested the entered ket with a regex which checks for a-z, A-Z and numbers between 0-9. If it doesn’t match this pattern, I’ve copied the entered key value to ignoredValue state.
I’ve also added some CSS for the styling part here:
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
padding: 14px;
}
.NPInputValueField {
padding: 6px 10px;
width: 200px;
font-size: 14px;
margin-top: 12px;
}
.NPInputResponseContainer {
margin: 14px 0px;
padding: 4px 8px;
border: 1px solid rgb(150, 150, 150);
background: #eee;
max-width: 300px;
}
</style>
Code language: HTML, XML (xml)
Here’s how the view should look like:
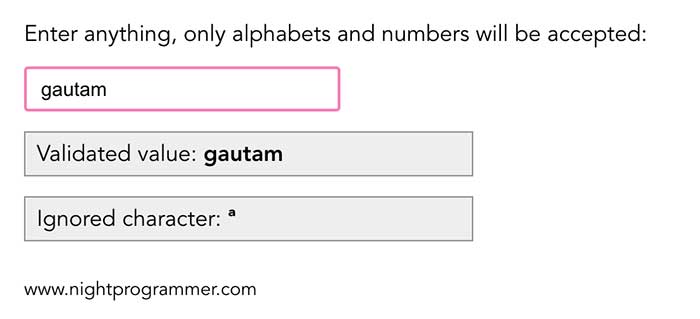
You can find a working demo of the above code from my repos below: