There are millions of ways to generate a random name in a JavaScript application. The NPM library is loaded with tons of many such libraries. Most of these libraries are based on CommonJS, which means, you cannot directly use them in your front-end application. There are, of course, libraries for the front-end as well. However, they’re often not as effective. Therefore, if you don’t want to look for a library and want to generate something random and unique on your own, you’re at the right place.
Another thing worth noticing is that most of the front-end libraries don’t use the system time as a medium of name generation. Time plays a crucial role in the generation of the name because no two instants of time are ever the same. Using time properly can guarantee uniqueness of 100% every time a name is generated. That is, no two names will ever be the same.
Before writing any code, you’d need the moment.js library to use a method. You can grab moment from NPM using:
npm install moment --save
Here’s the code I’ve written for the same:
<template>
<div id="app">
<div @click="generateRandomFilename()" class="np-button">
<div>Generate filename</div>
</div>
<div v-if="generatedRandomFilename" class="np-filename-container">
<div class="np-generate-title">Generated filename</div>
{{ generatedRandomFilename }}
</div>
<div class="np-credits">www.nightprogrammer.com</div>
</div>
</template>
<script>
import moment from "moment";
export default {
name: "App",
data() {
return {
generatedRandomFilename: null,
};
},
methods: {
generateRandomFilename() {
const randomString = this.generateRandomString(6);
const currentTimestamp = moment(new Date()).format(
"MM_DD_YYYY_h_mm_ss_SSS"
);
const randomNumber = Math.floor(Math.random() * 1000000);
const fileExtension = "";
this.generatedRandomFilename =
randomString +
"_" +
currentTimestamp +
"_" +
randomNumber +
fileExtension;
},
generateRandomString(stringLength) {
let result = "";
const alphaNumericCharacters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
const alphabetsLength = alphaNumericCharacters.length;
for (let i = 0; i < stringLength; i++) {
result += alphaNumericCharacters.charAt(Math.floor(Math.random() * alphabetsLength));
}
return result;
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
padding: 12px;
}
.np-button {
cursor: pointer;
background: #0059ff;
width: 160px;
text-align: center;
margin: 6px 0 20px 0;
color: #ffffff;
padding: 4px;
border-radius: 4px;
}
.np-credits {
font-size: 12px;
margin-top: 12px;
}
.np-generate-title {
font-weight: 600;
margin-bottom: 10px;
}
.np-filename-container {
border: 1px solid #0059ff;
border-radius: 4px;
padding: 16px;
max-width: 380px;
}
</style>
Code language: HTML, XML (xml)
Explanation
Let me explain the code a bit. When you click on the ‘Generate filename‘ button, the method on line 26 gets called. It creates a random string of 6 digits including a-z, A-Z and 0-9.
As you can see on line 47, I’m randomly picking 6 characters from the pool of the defined characters on line 43. I then return the result and store it on line 27, randomString constant.
On line 28, I’ve initialised the current time stamp using vanilla JavaScript’s new Date() method and passed it to the moment function. You’d notice that I’ve explicitly defined a format of “MM_DD_YYYY_h_mm_ss_SSS”. It expands as follows:
- MM: Month in number
- DD: Date in number
- YYYY: Year in number
- h: Hour
- MM: Minutes
- ss: Seconds
- SSS: Milliseconds with an accuracy of 3 digits
This SSS here is an important part of the uniqueness we need in the file name.
Then on line 31, I create a 6 digit random number.
On line 32, I define any file format if needed (“.pdf” for instance). I decided not to define any in this example.
Finally, the random name is generated on line 33 and is shown through the template code on line 8.
Here’s how the view should appear like:
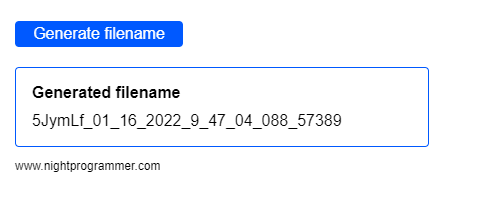
You can find a working version of the above demo from me repos here: